The 101 Introduction to Multimodal Deep Learning
Recent AI breakthroughs, like OpenAI’s GPT-4 Vision and Google’s Gemini 2.0, signal a shift toward multimodal deep learning—merging text, images, audio, and more into unified, context-rich systems.
GPT-4 Vision blends text and visual data for tasks like scene interpretation, while Gemini handles broader streams, including video and audio, showcasing multimodal versatility. By combining these data types, AI gains nuanced, human-like understanding, far surpassing unimodal limits.
This article will explore multimodal learning, why it’s important, how it works under the hood (from encoders to feature fusion), key training considerations, challenges to overcome, and real-world applications. We will also point to popular datasets and resources for diving deeper into multimodal learning.
To understand its scope, we first define the basics of multimodal learning.
What is Multimodal Learning in Deep Learning?
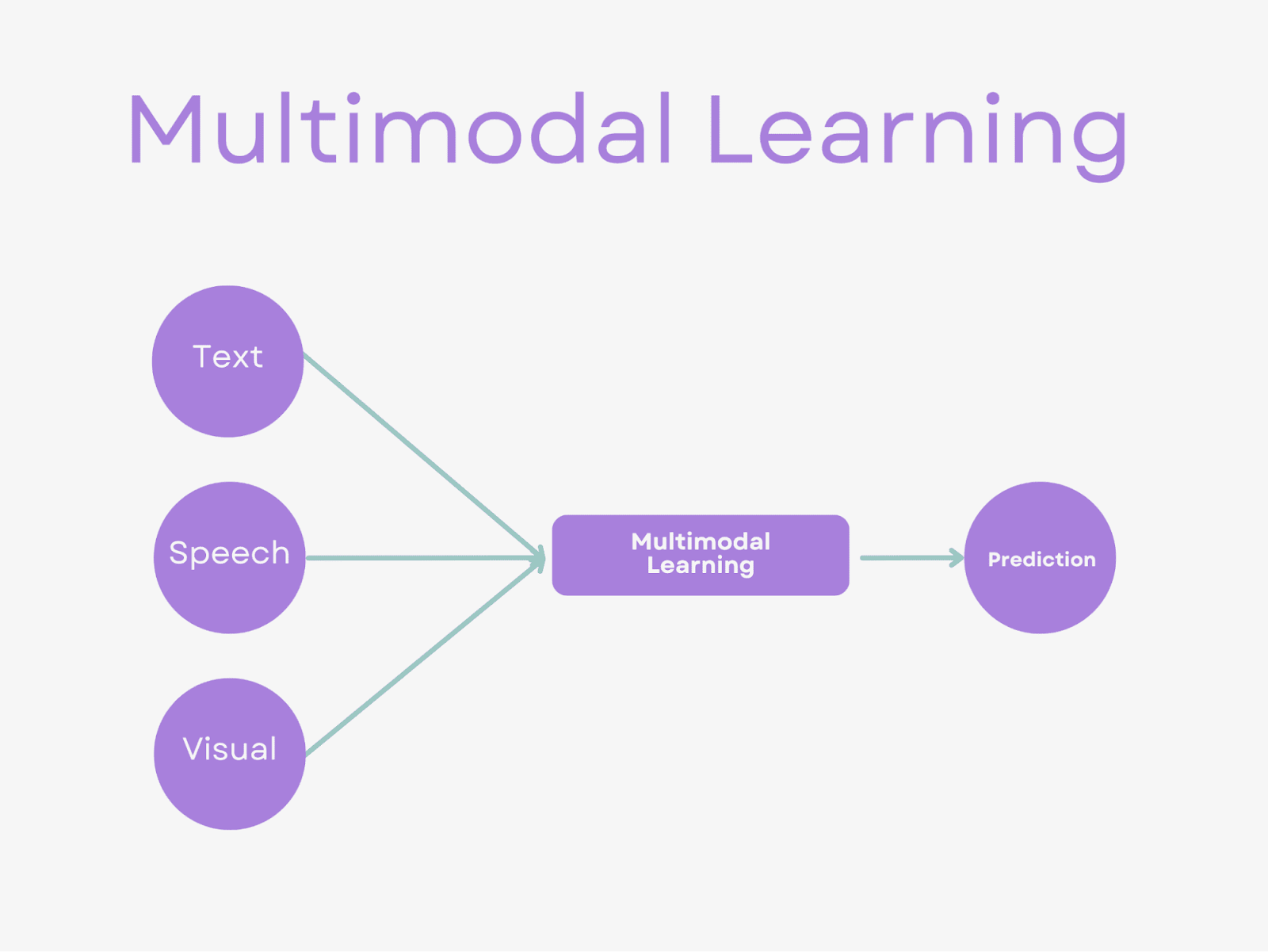
In multimodal deep learning, a model is trained on data that includes multiple modalities – different forms of information such as text, images, audio, and video.
Instead of learning from one input type alone, the model integrates information from various sources. The goal is to leverage the complementary strengths of each modality to make more accurate and comprehensive predictions.
For example, a multimodal system might analyze an image with a descriptive caption rather than treating it alone. By processing these together, the model can capture correlations (like matching spoken words to objects in a video) that would be invisible to a single-modality model.
Why is Multimodal Learning Important?
The importance of multimodal learning lies in its ability to address complex, real-world problems that are inherently multimodal. Many tasks require integrating information from various data types. For example:
- Visual Question Answering (VQA): Answering questions about images that requires both visual understanding and language comprehension.
- Image Captioning: Generating textual descriptions of images, combining vision and language.
- Emotion Recognition: Detecting emotions from video and audio, integrating visual cues (facial expressions) with auditory cues (tone of voice).
- Autonomous Driving: Processing data from cameras, LiDAR, and other sensors to navigate safely, which involves multiple modalities.
By leveraging multimodal data, these models can achieve higher accuracy and more robust performance than unimodal models, as they can capture complementary information that might be missing in a single modality.
Data Modalities in Multimodal Deep Learning
Multimodal learning relies on integrating distinct data modalities, commonly including:
- Text: Provides semantic information, capturing meaning and intent.
- Vision: Images and video offer spatial and visual context.
- Audio: Captures nuances in speech, sounds, and emotional tone.
Additionally, specialized modalities include:
- LiDAR offers precise depth and spatial data, critical in autonomous driving.
- Medical Imaging: Enhances diagnostic accuracy when combined with clinical records.
- Physiological Sensors: EEG and ECG data for comprehensive medical assessments.
Each modality brings unique strengths, contributing vital context. Images and video enrich spatial understanding; text provides semantic clarity, and audio complements emotional and situational nuances. Effectively merging these strengths allows multimodal models to achieve richer, more accurate representations, facilitating powerful and nuanced decision-making across applications.
A relevant video resource for understanding these modalities is available on YouTube Video, which explains multimodal data processing.
💡 Pro Tip: Master embeddings for multimodal fusion. See why they matter in the Importance of Embeddings guide.
How Multimodal Learning Works in Deep Learning
Multimodal learning involves several key steps, each critical for processing and integrating diverse data types:
Modality-Specific Encoders
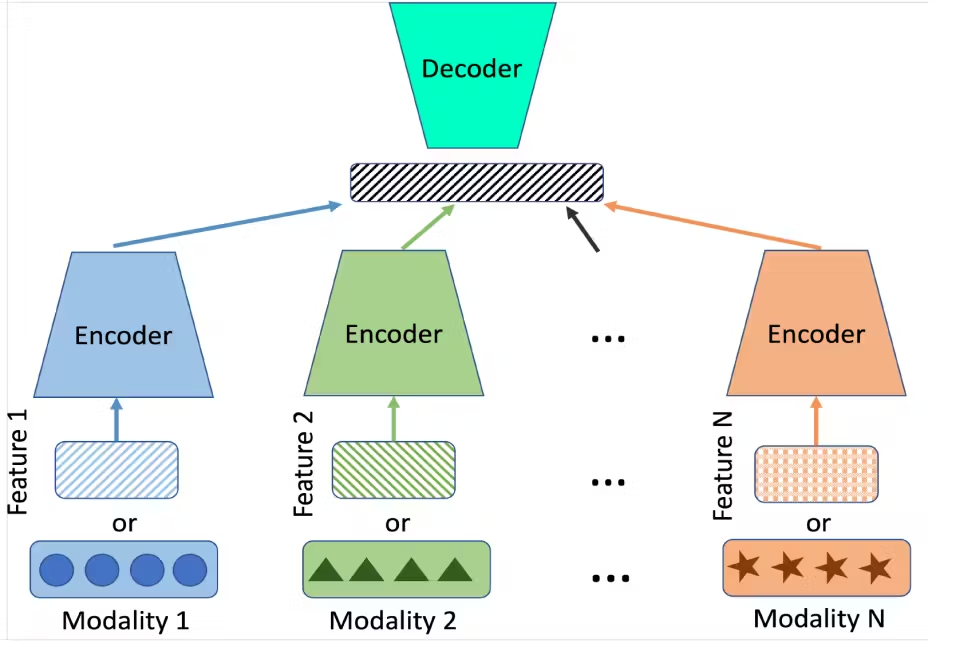
Multimodal models process each input modality with a dedicated encoder tailored to that data type. For instance, an image encoder might use a CNN like ResNet, while a text encoder could use a Transformer model like BERT.
These encoders extract features specific to each modality, ensuring that the unique characteristics of images, text, or audio are captured effectively. For example, ResNet might output a 2048-dimensional feature vector for an image, while BERT might produce a 768-dimensional embedding for a sentence.
The output of each encoder is a feature vector or set of features capturing the information in that modality. For example, an image encoder might output a 128-dimensional vector representing the image’s content, while a text encoder produces a similar vector for the semantic meaning of the text.
These embeddings are often projected into a shared latent space to facilitate fusion, ensuring that different modalities can be compared or combined. This alignment is crucial for tasks like cross-modal retrieval, where the model needs to match images with text descriptions.
Fusion Techniques
A multimodal model must fuse information from the different modalities after obtaining embeddings. There are several strategies:
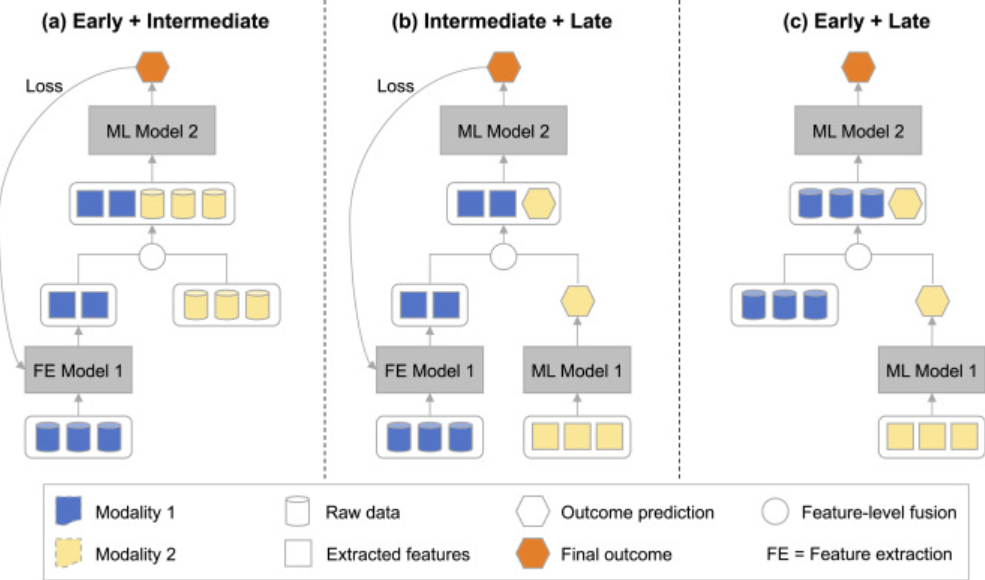
Early Fusion (Feature-Level Fusion)
Concatenate or combine the raw features or embeddings from each modality early in the model, then process them together. For example, an image feature vector and a text embedding vector can be concatenated into one long vector, which is then fed into subsequent layers jointly.
Early fusion preserves information from all modalities, allowing the network to learn cross-modal interactions immediately. It is essentially vector concatenation and ensures no modality is omitted initially. However, it may require careful preprocessing (to normalize scales of features) and may lead to very high-dimensional inputs, increasing computational costs.
Code Example
To illustrate, consider a simplified PyTorch model for a vision-language task, where an image is encoded with a CNN (ResNet) and text with a Transformer, then fused via early fusion for classification. This example, while basic, demonstrates how modalities are processed and combined, highlighting the practical implementation of these concepts:
import torch
import torch.nn as nn
import torchvision.models as models
from transformers import BertModel
class VisionLanguageEarlyFusion(nn.Module):
"""
Simple vision-language model using early fusion
- Image features from ResNet18
- Text features from BERT
- Early fusion by concatenation
"""
def __init__(self, num_classes=10):
super().__init__()
# Image encoder (ResNet18) - outputs 512-dim features
self.image_encoder = models.resnet18(pretrained=True)
self.image_encoder = nn.Sequential(*list(self.image_encoder.children())[:-1])
# Text encoder (BERT) - outputs 768-dim features
self.text_encoder = BertModel.from_pretrained('bert-base-uncased')
# Early fusion and classification
self.classifier = nn.Sequential(
nn.Linear(512 + 768, 256), # Concatenated features
nn.ReLU(),
nn.Dropout(0.2),
nn.Linear(256, num_classes)
)
def forward(self, images, input_ids, attention_mask):
# Process images
batch_size = images.size(0)
image_features = self.image_encoder(images)
image_features = image_features.view(batch_size, -1) # Flatten: [B, 512]
# Process text
text_outputs = self.text_encoder(input_ids=input_ids, attention_mask=attention_mask)
text_features = text_outputs.last_hidden_state[:, 0, :] # [CLS] token: [B, 768]
# Early fusion: concatenate features
fused_features = torch.cat([image_features, text_features], dim=1) # [B, 1280]
# Classification
output = self.classifier(fused_features)
return output
# Example usage
def main():
# Model initialization
model = VisionLanguageEarlyFusion(num_classes=10)
# Dummy inputs (for demonstration)
batch_size = 4
images = torch.randn(batch_size, 3, 224, 224) # [B, C, H, W]
input_ids = torch.randint(0, 30522, (batch_size, 128)) # [B, seq_len]
attention_mask = torch.ones(batch_size, 128) # [B, seq_len]
# Forward pass
with torch.no_grad():
outputs = model(images, input_ids, attention_mask)
print(f"Output shape: {outputs.shape}") # [B, num_classes]
if __name__ == "__main__":
main()
The code demonstrates:
- Image encoding using ResNet18 to extract visual features (512 dimensions)
- Text encoding using BERT to extract textual features (768 dimensions)
- Early fusion by concatenating these features (1280 dimensions total)
- A simple classifier that processes the fused features
For a more advanced model, hybrid fusion (discussed below) with cross-modal attention could be implemented, allowing the text encoder to attend to image features and enhancing cross-modal understanding.
Late Fusion (Decision-Level Fusion)
The late fusion technique processes each modality independently through its encoder and possibly modality-specific layers and only combines the outputs at the end. Each modality might produce a prediction (or a high-level feature) in a late fusion setup. Then, these predictions or features are combined (e.g., averaged, weighted, or through a small neural network) to produce the final output.
Late fusion allows each modality to be modeled in-depth, leveraging the strengths of each modality’s network without interference. It is also interpretable since one can inspect each modality’s output before fusion. The downside is that the model might learn to rely on the most predictive modality and ignore others until the final step. It doesn’t learn joint feature representations early on, potentially missing cross-modal relationships.
Hybrid Fusion (Mid-Level or Joint Fusion)
A combination of early and late fusion that merges modalities at intermediate layers and may allow multiple interaction points. One popular approach is using cross-modal attention: it allows the model to determine "which parts of modality A should I pay attention to, based on what I know from modality B?"
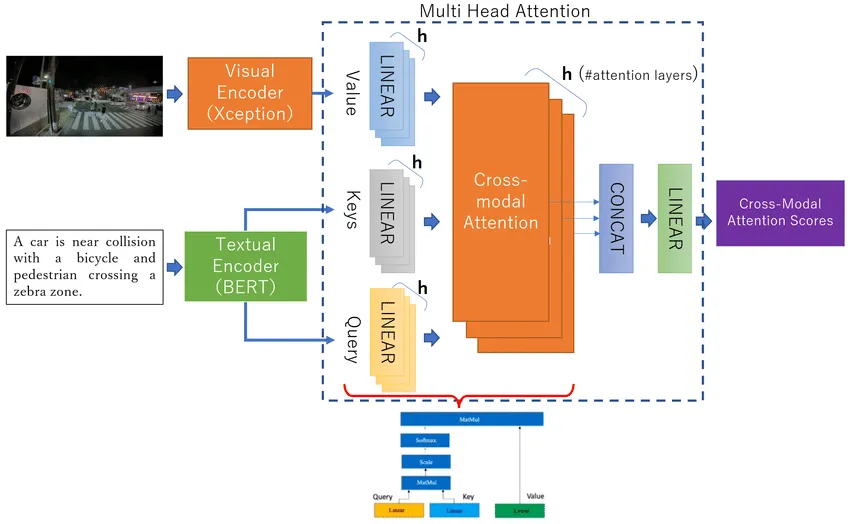
In this figure, cross-modal attention works by connecting visual and textual information through a query-key-value mechanism. The system calculates attention scores that represent the strength of connections between specific textual concepts (like "car" or "collision") and visual elements in the scene. After processing through multiple attention heads and linear transformations, the model produces cross-modal attention scores that reveal how strongly each textual element relates to different visual features.
Another hybrid approach is to project all modalities into a shared latent space (as in joint embedding models like CLIP) during training, effectively fusing by aligning representations rather than by direct concatenation.
Hybrid fusion aims to capture complex relationships but tends to make the model architecture more complex, requiring more computational resources and careful design.
Multimodal Model Training Considerations
Training multimodal models involves several considerations to ensure effective learning:
Loss Functions
Multimodal deep learning trains AI models on different data types, like images and text, using specific loss functions to measure errors and improve performance.
Cross-entropy loss is common for classification tasks, while contrastive losses are used for tasks like aligning image and text embeddings (e.g., in CLIP). Other losses, such as triplet loss for similarity learning, may be employed for multimodal retrieval tasks.
Contrastive losses, in particular, are crucial for tasks requiring alignment, such as ensuring that an image of a cat and the text "a cat" are mapped close together in the embedding space.
Data Alignment
Ensuring that data from different modalities is synchronized is crucial. For example, in video captioning, the timing of video frames must align with the corresponding text or audio. Misalignment can occur due to differences in sampling rates, sequence lengths, or inherent characteristics of the modalities (e.g., temporal differences between video frames and audio).
Data alignment ensures that different modalities are synchronized and their relationships are established, enabling effective fusion for downstream tasks. The table below summarizes the common data alignment techniques and their applications in multimodal deep learning.
Handling Imbalance
Modality imbalance, where one modality dominates in quantity or informativeness, can bias the model. Techniques like weighted sampling, modality dropout (randomly omitting a modality during training), or curriculum learning can help mitigate this issue, ensuring the model learns from all modalities effectively.
💡 Pro Tip: Are you struggling with training efficiency? Check out Knowledge Distillation Trends for techniques to streamline multimodal model training.